How we will do it: Connect to Google server, fetch the page header that contain the Timestamp and do some string manipulation to obtain the Date & Time information in the format that we need to be able to update our Real Time Clock:
getTime = function() conn=net.createConnection(net.TCP, 0) conn:on("connection",function(conn, payload) conn:send("HEAD / HTTP/1.1\r\n".. "Host: google.com\r\n".. "Accept: */*\r\n".. "User-Agent: Mozilla/4.0 (compatible; esp8266 Lua;)".. "\r\n\r\n") end) conn:on("receive", function(conn, payload) tstamp = string.sub(payload,string.find(payload,"Date: ")+6,string.find(payload,"Date: ")+35) --print('Timestamp : '..tstamp) conn:close() end) conn:connect(80,'google.com') conn = nil t = {} i=0 for token in string.gmatch(tstamp, "[^%s]+") do t[i] = token --print(t[i]) i=i+1 end wkd = {["Sun"]=1, ["Mon"]=2, ["Tue"]=3, ["Wed"]=4, ["Thu"]=5, ["Fri"]=6, ["Sat"]=7 } mnt = {["Jan"]=1, ["Feb"]=2, ["Mar"]=3, ["Apr"]=4, ["May"]=5, ["Jun"]=6, ["Jul"]=7, ["Aug"]=8, ["Sep"]=9, ["Oct"]=10, ["Nov"]=11,["Dec"]=12} wk1=string.sub(t[0],0,3) dtd=wkd[wk1] d1=t[1] mn1=t[2] mnl=mnt[mn1] y1=string.sub(t[3],3,4) h1=2+string.sub(t[4],0,2) m1=string.sub(t[4],4,5) s1=string.sub(t[4],7,8) --print("Date : "..wk1..", "..d1.."/"..mn1.."/"..t[3]) --print("Time : "..h1..":"..m1..":"..s1) return s1,m1,h1,d1,dtd,mnl,y1 end
For testing, pack it together and save the code on ESP as ‘gettime.lua‘, restart ESP and run:
--GetTime from Google Server and set RTC Time require('gettime') s2,m2,h2,d2,dtd2,l2,y2=getTime() print("Date : "..dtd..", "..d1.."/"..mn1.."/"..y1) print("Time : "..h1..":"..m1..":"..s1) -- Set Time and Date require('pcf8563') sda, scl = 2, 1 pcf8563:init(sda, scl) pcf8563:setTime(s1,m1,h1,d1,dtd,mnl,y1)
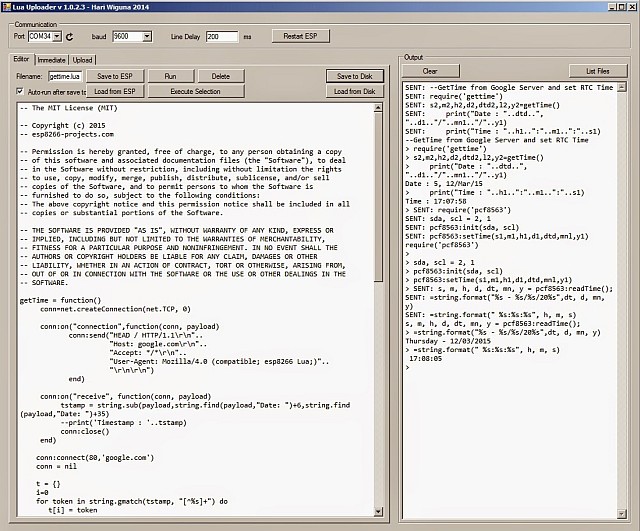
If you run in trouble because of lack of memory, before running, just compile the programs, it will help a lot:
node.compile("gettime.lua") node.compile("pcf8563.lua")